首页
NodeMcu
NodeMcu_ESP8266开发板--TCP Server
NodeMcu_ESP8266简介
NodeMcu_温湿度传感器(DHT11)的使用
esp8266+DHT11温湿传感器 制作web室内温度计
ESP8266NodeMcu网页控制四个LED灯
NodeMcu用网页上传图片来驱动1.44寸TFT屏幕
esp8266使用1.44寸tft屏Arduino模块示例程序
1.44寸TFT屏ST7735使用方法
NodeMcu_ESP8266编程
NodeMcu环境监控+远程控制
NodeMcu实现网页远程控制+温湿度显示
NodeMcu网页控制+温湿度显示+TFT
NodeMcu、ESP8266、ESP32区别
本文档由 内网文摘 发布,转载请注明出处
-
+
首页
1.44寸TFT屏ST7735使用方法
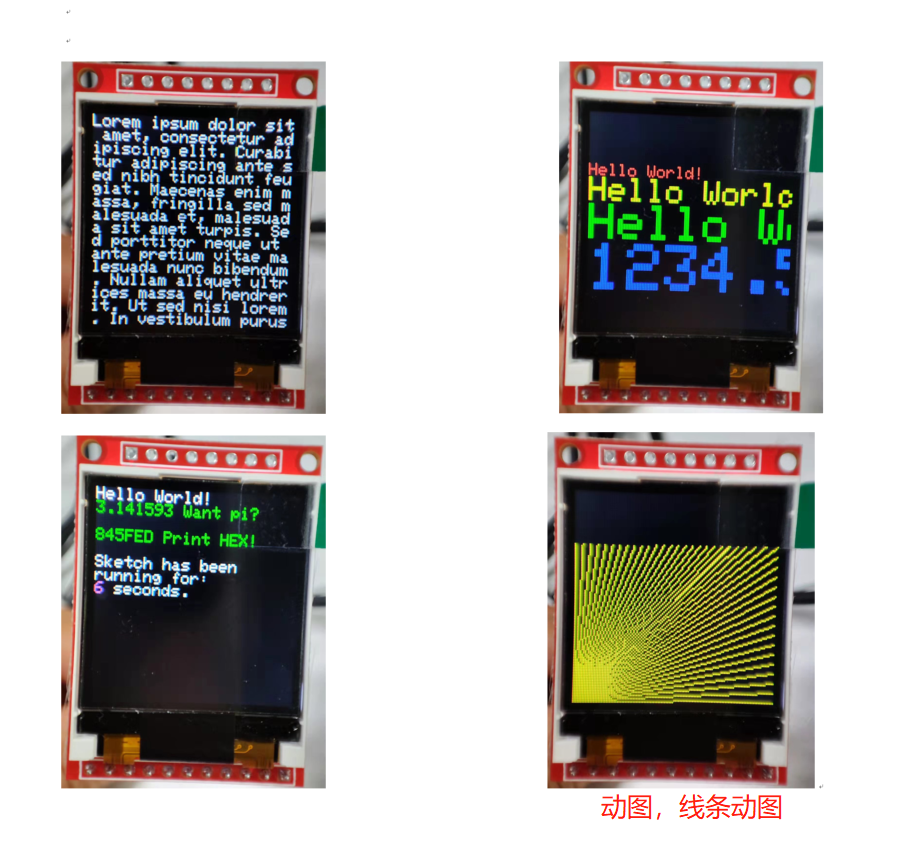 参考: [Arduin-ST7735](https://blog.csdn.net/zuoheizhu/article/details/90671052 "Arduin-ST7735") [Arduino开发板使用TFT LCD液晶显示屏的终极新手入门指南](https://www.yiboard.com/thread-1060-1-1.html "Arduino开发板使用TFT LCD液晶显示屏的终极新手入门指南") 需要用到2个库,分别是:Adafruit的GFX库和Adafruit的ST7735 本文实现了,矩形,复杂多边形,文字,颜色等,绘制于显示 **注:** - 屏幕左上角为原点,坐标为0,0; - DC引脚,即RS引脚; ### 函数使用 参考资料:https://wiki.microduino.cn/index.php/Microduino-Module_TFT_Reference ### Constructor/结构体 Adafruit_ST7735() #### 显示文字示例 ```cpp #include <Adafruit_GFX.h> // Core graphics library #include <Adafruit_ST7735.h> // Hardware-specific library #include <SPI.h> // For the breakout, you can use any 2 or 3 pins // These pins will also work for the 1.8" TFT shield #define TFT_CS D1 #define TFT_RST D2 // you can also connect this to the Arduino reset #define TFT_DC D3 Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST); void setup(void) { tft.initR(INITR_144GREENTAB); //初始化ST7735S芯片,黑色标签 tft.fillScreen(ST7735_BLACK); //填充背景颜色(必须填充,否则之前的图像,将不会消失) tft.setCursor(10, 40); tft.setTextColor(ST7735_YELLOW); tft.setTextSize(0); tft.println("Hello World!"); } void loop(){ } ``` ### Constants/常量 #### 预定义颜色 ```cpp #define ST7735_BLACK 0x0000 #define ST7735_BLUE 0x001F #define ST7735_RED 0xF800 #define ST7735_GREEN 0x07E0 #define ST7735_CYAN 0x07FF #define ST7735_MAGENTA 0xF81F #define ST7735_YELLOW 0xFFE0 #define ST7735_WHITE 0xFFFF ``` ### Functions/函数 #### 图形 ```cpp //用颜色填充整个屏幕 //tft.fillScreen(uint16_t color) //参数 color:填充的颜色 tft.fillScreen(ST7735_YELLOW); //在TFT屏幕上画出一条横线 //tft.drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color) //x:起始点x轴坐标;y:起始点y轴坐标;w:横线长度;color:线的颜色 tft.drawFastHLine(10, 80, 100, ST7735_YELLOW); //在TFT屏幕上画出一条竖线 //tft.drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color) //x:起始点x轴坐标;y:起始点y轴坐标;w:竖线长度;color:线的颜色 tft.drawFastVLine(60, 20, 100, ST7735_RED); //在TFT屏幕上画出一条任意角度的直线 //tft.drawLine(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color) //参数 x0:起始点x轴坐标; y0:起始点y轴坐标; x1:终止点x轴坐标; y1:终止点y轴坐标; color:线的颜色 tft.drawLine(10, 20, 80, 150, ST7735_GREEN); //在TFT屏幕上画出一个方形轮廓 //tft.drawRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color) //参数 x:起始点x轴坐标; y:起始点y轴坐标; w:方形长长度; ; h:方形宽长度; color:方形的颜色 tft.drawRect(20, 50, 40, 20, ST7735_BLUE); //在TFT屏幕上画出一个实心方形 //tft.fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color) //参数 x:起始点x轴坐标; y:起始点y轴坐标; w:方形长长度; h:方形宽长度; color:方形的颜色 tft.fillRect(20, 50, 40, 20, ST7735_BLUE); //在TFT屏幕上画出一个圆形轮廓 //tft.drawCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) //参数 x0:圆心的x轴坐标; y0:圆心的y轴坐标; r:圆的半径; color:圆形的颜色 tft.drawCircle(40, 40, 20, ST7735_YELLOW); //在TFT屏幕上画出一个实心圆 //tft.fillCircle(int16_t x0, int16_t y0, int16_t r, uint16_t color) //参数 x0:圆心的x轴坐标; y0:圆心的y轴坐标; r:圆的半径; color:实心圆形的颜色 tft.fillCircle(40, 40, 20, ST7735_YELLOW); //在TFT屏幕上画出一个三角形轮廓 //tft.drawTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) //参数 x0:三角形顶点x轴坐标; y0:三角形顶点y轴坐标; x1:三角形左下点x轴坐标; y1:三角形左下点y轴坐标 // x2:三角形右下点x轴坐标; y2:三角形右下点y轴坐标; color:三角形的颜色 tft.drawTriangle(43, 30, 40, 90, 80, 120, ST7735_RED); //在TFT屏幕上画出一个实心三角形 //tft.fillTriangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color) //参数 x0:三角形顶点x轴坐标; y0:三角形顶点y轴坐标; x1:三角形左下点x轴坐标; y1:三角形左下点y轴坐标 //x2:三角形右下点x轴坐标; y2:三角形右下点y轴坐标; color:实心三角形的颜色 tft.fillTriangle(43, 30, 40, 90, 80, 120, ST7735_RED); //在TFT屏幕上画出一个圆角方形轮廓 //tft.drawRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, int16_t radius, uint16_t color) //参数 x0:起始点x轴坐标; y0:起始点y轴坐标; w:圆角方形长长度; h:圆角方形宽长度; //radius:圆角方形圆角半径; color:圆角方形的颜色 tft.drawRoundRect(30, 40, 60, 65, 20, ST7735_GREEN); //在TFT屏幕上画出一个实心圆角方形 //tft.fillRoundRect(int16_t x0, int16_t y0, int16_t w, int16_t h, int16_t radius, uint16_t color) //参数 x0:起始点x轴坐标; y0:起始点y轴坐标; w:圆角方形长长度; h:圆角方形宽长度; //radius:圆角方形圆角半径; color:实心圆角方形的颜色 tft.fillRoundRect(30, 40, 60, 65, 20, ST7735_GREEN); ``` #### 文字 ```cpp //设置在TFT屏幕上打印文字的起始点坐标 //tft.setCursor(int16_t x, int16_t y) //参数 x:起始点x轴坐标; y:起始点y轴坐标 tft.setCursor(10, 40); //设置在TFT屏幕上打印文字的颜色 //tft.setTextColor(uint16_t c) //参数 color:文字颜色 tft.setTextColor(ST7735_YELLOW); //设置在TFT屏幕上打印文字的字号 //tft.setTextSize(uint8_t s) //参数 s:文字字号0/1/2 tft.setTextSize(0); //设置在TFT屏幕上打印文字的内容 //tft.println(" ") //参数 引号里写打印的内容 tft.println("Microduino!"); ``` #### 其它 ```cpp //显示屏的分辨率 tft.width(); //int16_t width(void); tft.height(); //int16_t height(void); tft.fillScreen(ST7735_BLACK);//填充屏幕,为黑色(必须填充,否则之前的图像,将不会消失) tft.setTextColor(ST7735_WHITE);//设置字体颜色,为白色 tft.setTextSize(0); //设置字体大小为,0 tft.println("Hello World!"); tft.setTextColor(ST7735_GREEN);//设置字体颜色,为绿色 float p = 3.1415926; //float是浮点型数据类型 tft.print(p, 6); //显示,p这个变量,第6位以后,不再显示 tft.print(8675309, HEX); //以十六进制打印8675309 //就只是在,屏幕正中间画了一个点(打印一个像素) tft.drawPixel(tft.width()/2, tft.height()/2, ST7735_GREEN); tft.fillScreen(ST7735_BLACK);//填充屏幕,为黑色(必须填充,否则之前的图像,将不会消失) tft.setCursor(0, 0);//设置光标的起始位置 tft.setTextColor(ST7735_YELLOW);//设置字体颜色,为黄色 tft.setTextWrap(true); //文本自动换行 tft.print(text); ``` ### 源码 ```cpp #include <Adafruit_GFX.h> // Core graphics library #include <Adafruit_ST7735.h> // Hardware-specific library #include <SPI.h> //这些引脚也适用于1.8英寸TFT #define TFT_CS D1 #define TFT_RST D2 // 您也可以将其连接到Arduino重置 #define TFT_DC D3 #define TFT_BACKLIGHT D4 //显示屏背光销 //从Adafruit_ST7735类创建一个名为TFT的对象,并在LCD和Arduino之间提供SPI通信 //对于1.44“和1.8”TFT与ST7735(包括HalloWing)使用: Adafruit_ST7735 tft = Adafruit_ST7735(TFT_CS, TFT_DC, TFT_RST); float p = 3.1415926; void setup(void) { Serial.begin(9600); Serial.print("Hello! ST7735 TFT Test"); tft.initR(INITR_144GREENTAB); //初始化ST7735S芯片,黑色标签 pinMode(TFT_BACKLIGHT, OUTPUT);//定义背光 digitalWrite(TFT_BACKLIGHT, HIGH);//设置背光,D4默认拉高可不设置 Serial.println("Initialized"); uint16_t time = millis(); tft.fillScreen(ST7735_BLACK); time = millis() - time; Serial.println(time, DEC); delay(500); //大块文字 tft.fillScreen(ST7735_BLACK); testdrawtext("123456", ST7735_WHITE); //其中WHITE,定义字体为白色 delay(1000); // tft打印功能! tftPrintTest(); delay(4000); //一个像素 tft.drawPixel(tft.width()/2, tft.height()/2, ST7735_GREEN); //就只是在,屏幕正中间画了一个点 delay(500); //线条绘制测试 testlines(ST7735_YELLOW); delay(500); //优化的线条 testfastlines(ST7735_RED, ST7735_BLUE); delay(500); testdrawrects(ST7735_GREEN); delay(500); testfillrects(ST7735_YELLOW, ST7735_MAGENTA); delay(500); tft.fillScreen(ST7735_BLACK); testfillcircles(10, ST7735_BLUE); testdrawcircles(10, ST7735_WHITE); delay(500); testroundrects(); delay(500); testtriangles(); delay(500); mediabuttons(); delay(500); Serial.println("完成"); delay(1000); } void loop() { tft.invertDisplay(true); delay(500); tft.invertDisplay(false); delay(500); } void testlines(uint16_t color) { tft.fillScreen(ST7735_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(0, 0, x, tft.height()-1, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(0, 0, tft.width()-1, y, color); delay(0); } tft.fillScreen(ST7735_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(tft.width()-1, 0, x, tft.height()-1, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(tft.width()-1, 0, 0, y, color); delay(0); } tft.fillScreen(ST7735_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(0, tft.height()-1, x, 0, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(0, tft.height()-1, tft.width()-1, y, color); delay(0); } tft.fillScreen(ST7735_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(tft.width()-1, tft.height()-1, x, 0, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(tft.width()-1, tft.height()-1, 0, y, color); delay(0); } } void testdrawtext(char *text, uint16_t color) { tft.setCursor(0, 0); tft.setTextColor(color); tft.setTextWrap(true); tft.print(text); } void testfastlines(uint16_t color1, uint16_t color2) { tft.fillScreen(ST7735_BLACK); for (int16_t y=0; y < tft.height(); y+=5) { tft.drawFastHLine(0, y, tft.width(), color1); } for (int16_t x=0; x < tft.width(); x+=5) { tft.drawFastVLine(x, 0, tft.height(), color2); } } void testdrawrects(uint16_t color) { tft.fillScreen(ST7735_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color); } } void testfillrects(uint16_t color1, uint16_t color2) { tft.fillScreen(ST7735_BLACK); for (int16_t x=tft.width()-1; x > 6; x-=6) { tft.fillRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color1); tft.drawRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color2); } } void testfillcircles(uint8_t radius, uint16_t color) { for (int16_t x=radius; x < tft.width(); x+=radius*2) { for (int16_t y=radius; y < tft.height(); y+=radius*2) { tft.fillCircle(x, y, radius, color); } } } void testdrawcircles(uint8_t radius, uint16_t color) { for (int16_t x=0; x < tft.width()+radius; x+=radius*2) { for (int16_t y=0; y < tft.height()+radius; y+=radius*2) { tft.drawCircle(x, y, radius, color); } } } void testtriangles() { tft.fillScreen(ST7735_BLACK); int color = 0xF800; int t; int w = tft.width()/2; int x = tft.height()-1; int y = 0; int z = tft.width(); for(t = 0 ; t <= 15; t++) { tft.drawTriangle(w, y, y, x, z, x, color); x-=4; y+=4; z-=4; color+=100; } } void testroundrects() { tft.fillScreen(ST7735_BLACK); int color = 100; int i; int t; for(t = 0 ; t <= 4; t+=1) { int x = 0; int y = 0; int w = tft.width()-2; int h = tft.height()-2; for(i = 0 ; i <= 16; i+=1) { tft.drawRoundRect(x, y, w, h, 5, color); x+=2; y+=3; w-=4; h-=6; color+=1100; } color+=100; } } void tftPrintTest() { tft.setTextWrap(false); //设置字体包裹,是否打开 tft.fillScreen(ST7735_BLACK); //填充背景颜色(必须填充,否则之前的图像,将不会消失) tft.setCursor(0, 30); //设置游标初始位置,x轴为0,Y轴为30 tft.setTextColor(ST7735_RED); //设置字体为红色 tft.setTextSize(1); tft.println("Hello World!"); tft.setTextColor(ST7735_YELLOW); tft.setTextSize(2); tft.println("Hello World!"); tft.setTextColor(ST7735_GREEN); tft.setTextSize(3); tft.println("Hello World!"); tft.setTextColor(ST7735_BLUE); tft.setTextSize(4); tft.print(1234.567); delay(1500); tft.setCursor(0, 0);//设置光标的起始位置 tft.fillScreen(ST7735_BLACK);//填充屏幕,为黑色(必须填充,否则之前的图像,将不会消失) tft.setTextColor(ST7735_WHITE);//设置字体颜色,为白色 tft.setTextSize(0); //设置字体大小为,0 tft.println("Hello World!"); tft.setTextSize(1); tft.setTextColor(ST7735_GREEN); tft.print(p, 6); //显示,p这个变量,第6位以后,不再显示 tft.println(" Want pi?"); tft.println(" "); tft.print(8675309, HEX); // print 8,675,309 out in HEX! tft.println(" Print HEX!"); tft.println(" "); tft.setTextColor(ST7735_WHITE); tft.println("Sketch has been"); tft.println("running for: "); tft.setTextColor(ST7735_MAGENTA); tft.print(millis() / 1000); tft.setTextColor(ST7735_WHITE); tft.print(" seconds."); } void mediabuttons() { // play tft.fillScreen(ST7735_BLACK); tft.fillRoundRect(25, 10, 78, 60, 8, ST7735_WHITE); tft.fillTriangle(42, 20, 42, 60, 90, 40, ST7735_RED); delay(500); // pause tft.fillRoundRect(25, 90, 78, 60, 8, ST7735_WHITE); tft.fillRoundRect(39, 98, 20, 45, 5, ST7735_GREEN); tft.fillRoundRect(69, 98, 20, 45, 5, ST7735_GREEN); delay(500); // play color tft.fillTriangle(42, 20, 42, 60, 90, 40, ST7735_BLUE); delay(50); // pause color tft.fillRoundRect(39, 98, 20, 45, 5, ST7735_RED); tft.fillRoundRect(69, 98, 20, 45, 5, ST7735_RED); // play color tft.fillTriangle(42, 20, 42, 60, 90, 40, ST7735_GREEN); } ```
local
2021年10月29日 15:30
分享文档
收藏文档
上一篇
下一篇
微信扫一扫
复制链接
手机扫一扫进行分享
复制链接
关于 LocalNetwork
LocalNetwork
是由mrdoc开源
LocalNetwork.cn
修改的在线文档系统,作为个人和小型团队的云笔记、文档和知识库管理工具。
如果此文档给你或你的团队带来了帮助,欢迎支持作者持续投入精力更新和维护!内网文摘 & LocalNetwork
>>>主页
logo
logo
下载Markdown文件
分享
链接
类型
密码
更新密码